A brief history of the web and javascript
This is a small article about the history of the web and the JavaScript language. The goal is to provide context and understanding to the modern jungle of web development tools and frameworks — how they came to be and why we use them.
So, ever wondered how the Web actually works and how it came to be? Why we use bundlers in JavaScript? or why meta-frameworks is a thing? Then this is for you. Hope you’ll enjoy it!
1. How and why the web was created
There were three circumstances that resulted in the creation of the Web. First, research on networking and package-based transmission had reached a state where the TCP/IP stack had been invented and we saw the early stages of the internet being built. Secondly, for some time there had been ongoing research on Hypertext, which gives the ability to display digital documents and link between them via hyperlinks . Thirdly, there was a need for a system for organizing and keeping track of documents at CERN, since they were loosing track of useful documents when projects completed and researchers left the organization.
The main person behind the web was Tim Berner-Lee, and the time of its conception was at the end of the 1980’s. Tim, who worked at CERN at the time, had now had several experiences that gave him the idea for the web. In 1980, he had worked at CERN on a software system called ENQUIRE, made for organizing and accessing documents as Hypertext. He had then left CERN to work at John Poole's Image Computer Systems, where he learned about computer networking. He was now back at CERN for a fellowship and had a simple idea that would change the world: to combine networking concepts with Hypertext documents to create a distributed document system. This idea evolved into the system he called the World Wide Web (WWW).
I just had to take the hypertext idea and connect it to the TCP and DNS ideas and — ta-da!— the World Wide Web.
- Tim Berner-Lee
WWW had the following components:
- HyperText Markup Language (HTML). A language for writing HyperText documents that the browser could render to display the document graphically. These documents were also known as web pages, since they were accessed through The Web.
- HyperText Transport Protocol (HTTP). HTTP is built on top of the TCP/IP protocol stack, and is used to communicate between a browser and the web server over the internet. It was made specifically for requesting and sending hypertext documents. The browser would send a HTTP request for a document (a web page formatted as HTML) that is located on the web server, and the server would respond, using HTTP, with a message that contained the HTML for the web page.
- The web server. A software that listens to incoming HTTP requests on the network and handles them in a standardized way. The purpose of a web server is to process an incoming request and producing a fitting HTTP response, which is generally a message containing an HTML page.
- The browser. The access portal to the web. The browser is a software that translates a user entered web address or a click on a link, to a request to the web server, and which then renders the HTML response graphically to the user as a web page.
- The URI. The formatting of addresses to resources on the web. E.g., www.somedomain.com/someresource
One of the first browsers were violaWWW, initially released in 1991/1992, and was the recommended browser at CERN. Even this early browser had features like bookmarks and forward/backward browsing.
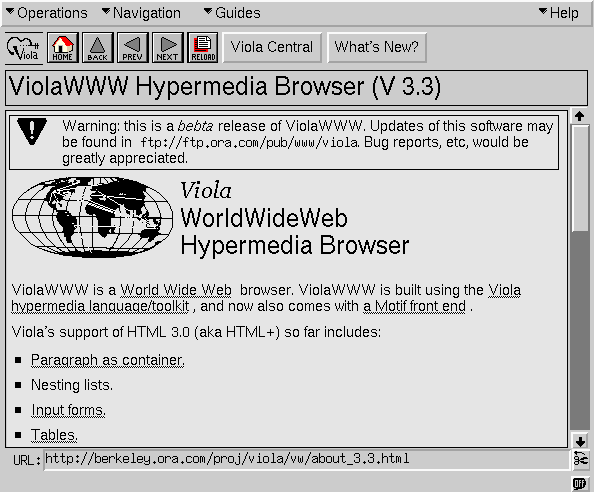
The first version of the Web (HTML, HTTP, Browser, Web server) was released outside CERN in 1991, and within the next two years, about 50 websites had been created 1. In 1993, CERN made the Web protocol and and code available for free, which enabled widespread use. Within less than a year, another browser called Mosaic was released, and thousands of websites had been created. There was now a large amount of web servers getting connected into the internet, creating the web of servers that Tim envisioned.
Up to this point, web pages were static once loaded, and the styling you could do was minimal. It was limiting, and developers wanted a way to style their web pages and use dynamic behavior, thus JavaScript (JS) and Cascading Style Sheets (CSS) was born in 1995 and 1996, respectively. JS for creating dynamic interaction with the website, and CSS to style them. These two languages were also, like HTML, made specifically to be rendered in the browser, and could not run elsewhere.
You now had three languages to use for developing web pages: HTML for the structure and content of the web page, JavaScript for creating interactivity, and CSS for styling.
2. How JavaScript became what it is today
This chapter is about understanding the complex landscape of JavaScript frameworks and tools. We'll explore JavaScript's history and give context to the modern state of JS.
The early days of JavaScript
The first version of JavaScript came in 1994. It was created at Netscape, which created one of the early browsers, to give websites dynamic behavior. JavaScript could only run inside the browser. In spite of its name, it had no association to Java.
Some of the important features of JavaScript:
- Access to the Document Object Model (DOM). When a web page is loaded in the browser, the browser creates an object-based representation of the page. JavaScript can access this to dynamically change the web page’s structure, style and content.
- Access to the Window object. This object represents a window in a browser containing a DOM document (a tab, window or iframe).
- An interpreted language with first-class functions. This means that functions are treated like variables. You can pass them around and call them only when needed.
- Single-threaded and based on asynchronous callbacks and events.
- Runs in the browser of the client. The server only supplies the client with the code, the clients browser then has to run it together with the webpage.
So web pages became dynamic and interactive. Great! But then there was a problem: Different browsers started implementing different JavaScript runtimes, supporting different parts of the JavaScript specification. This imposed the need to modify your code for different browsers if you wanted the web page to behave the same in both. (CSS and HTML had the same problem.)
Enter jQuery in 2006.
jQuery was created to simplify JavaScript and handle the different runtimes in different browsers. With jQuery you could use one function call and behind the scenes jQuery would implement the different implementations needed for each browser. jQuery also simplified the syntax, making it easier for the developer to program with JavaScript. jQuery is still widely used to this day.
JavaScript becomes general purpose, and bundlers becomes a thing
Then, in 2009, Ryan Dahl had a though: wouldn’t it be great to be able to run JavaScript outside of the browser? so he created Node.js, which is a runtime environment for running JavaScript outside of the browser, making it possible to use JavaScript as a general purpose programming language. Thus, JavaScript was no longer contained to the browser.
But, as all general-purpose languages, Node.js needed a way to import packages and modules. So Node.js introduced the CommonJS specification, a set of conventions for handling modules in JavaScript. So while in the browser you have to import explicit JavaScript files into the HTML document (and was unable to import modules within those JavaScript files), with the Node.js runtime you could import JavaScript modules directly into JavaScript files (just like other general purpose languages):
// CommonJS made modules possible
// Create a module by exporting one or more functions from a JavaScript file:
exports.uppercase = (str) => str.toUpperCase()
// import that module/file into another JavaScript and use its exported functions:
const uppercaseModule = require('uppercase.js')
uppercaseModule.uppercase('test')
The module system was so great that people wanted it for the browser too. And this is the main purpose of Bundlers. Bundlers compile your modularized JavaScript files into one single JavaScript file, a “bundle” file, that can then be imported into your HTML document. These bundlers, among other things, also provides code minimization and optimization, making your code run faster.
I was unable to find out which bundler came first, but Browserify, which came in 2011, were the oldest bundler I found. Webpack, which is the most popular bundler today, was first released in 2014.
So now you could import modules (or packages), and thus you could make modules public for others to import and use. To manage modules for node.js, npm was created, a package manager for node.
The era of Single-Page web Applications
Aside from creating simple dynamic behaviors, JavaScript also made it possible to develop applications for the web. (Applications is a general term for highly interactive websites with states and a dynamic UI.)
But creating apps with JavaScript is cumbersome and verbose, and it is hard to maintain large code bases. That is why client-side web frameworks emerged. These provided a standardized way to develop applications for the web. These frameworks allow you to write more declarative code – and sometimes less code overall, reducing the cognitive load of having to solve common tasks yourself. They had features such as:
- Reactive states. If you updated a piece of data, the framework would update the UI for you.
- Components. Being able to abstract different parts of a web site into components, that can be maintained alone and reused with other components to form a website or app. Organization of your code is a lot simpler.
- Single-page-application (SPA) architecture. SPAs do not fetch and render new HTML files — they load the whole application at the first request. They use single HTML shell that they continually update through JavaScript. Each pseudo-page in the app is loaded from data stored in JavaScript rather than making requests to addresses on the web. This decreases latency on the client side (except for the initial load), because you don't have to make a request to the server when you want to navigate to another page or update the UI.
While there are many of these front-end frameworks, three popular ones you may have heard of are Vue, React and Angular. The earliest frameworks I could find was Backbone and AngularJS, both being released in 2010. These frameworks were initially made for complex and large applications, but they can also aid with development for small applications, at least after you have overcome the initial learning-curve.
Meta-frameworks - A framework of a framework
But the SPA architecture came at a cost. The Search Engine Optimization (SEO) was thrown in the dirt, since all the search engine crawler sees when requesting your website is an empty HTML shell. Meta frameworks was created to deal with the SEO problems of SPAs. Examples are Angular Universal (for Angular), Nuxt (for Vue) and Next.js (for React). The earliest meta-frameworks seems to have been released around 2016.
These meta frameworks are specifically applied to SPA frameworks, and they do the following:
- In meta frameworks, views (pages) can be rendered on the server before being sent to the browser. This is called Server-Side Rendering (SSR). This provides more control over where pages were rendered. The pages that needed SEO can be rendered on the server, while those that do not need SEO can be rendered on the client side (faster).
- Additional convenience functions, structure, and hooks are added to provide a framework for creating so called “hybrid” apps: apps that are sometimes loaded on the client side, and sometimes loaded on the server side, usually dependent on if the page requires SEO.
- Static Site Generation (SSG). Most of these metaframeworks provides tooling to generate a static version of your app (a set of static HTML, CSS and JS files). These are faster to load and can be cached on CDN servers for faster latency. This is often used for sites which are not updated often, such as apps with “static” data, blogs, and so on.
So, just to clarify, what is the difference between meta frameworks and full-scale server-side frameworks such as Django or Express?
- Meta frameworks are made specifically for a specific frontend framework (Nuxt for Vue, Next.js for React, Angular Universal for Angular), to make these frontend frameworks perform well with SEO and to provide additional convenience for the developers. Altough the complexity of having to deal with learning a framework of a framework is not really convenient.
- SSR in meta frameworks generally happen at page load, not at specific requests made on-demand by the user.
- Server-side frameworks like Django or Express are made to create full-scale server-side backends, with HTTP REST APIs, authentication, database, and so on. To an extent, this can be done in meta frameworks, but not as well.
In modern apps, a common option is to use a meta framework for frontend and SEO, and then cloud services like Google Firebase for a server-less backend. This way you don’t have to manage your own servers.
Typescript: Making it easier to deal with large JavaScript codebases
The first public version of Typescript came in 2012 and was created by Microsoft. It originated from the shortcomings of JavaScript for the development of large-scale applications both at Microsoft and among their external customers.
TypeScript is basically a compiled version of JavaScript with syntax for writing strongly typed programs. It helps you write consistent code that is less likely to have bugs, which is likely to creep in when writing large-scale applications in normal JavaScript.
This only touches the surface, but a few important Typescript features and properties are:
- TypeScript adds syntax for types. Strict mode can be enabled such that types are declared for all functions and variables. TypeScript adds additional types such as interfaces (struct-like) and enums.
- Typescript transpiles to JavaScript by a compiler. Errors and inconsistencies can therefore be checked for at compile time rather than runtime, and you can discover problems with your code early.
- Better error-detection in the editor compared to JavaScript.
- Typescript is a superset of JavaScript, meaning that existing JavaScript programs are also valid TypeScript programs.
If you are planning to write a large application, TypeScript can be a good choice. It is widely used in large organizations.
State of JavaScript
JavaScript is standardized through ECMAscript standard, which first appeared in 1997 and designed by Brendan Eich at Netscape. The current version is the 13th edition of ECMA-262. The ECMAscript standard is managed and developed by tc39, a group of JavaScript developers, implementers, academics, and more, which collaborate with the community to maintain and evolve the definition of JavaScript.
It is the browsers job to decide what to implement from this standard, and of course they prioritize differently. A good website for seeing what features that are implemented in each browser is caniuse.com.
The frontend- and hybrid frameworks are evolving rapidly. There is a big competitions between these frameworks and every year they release new “groundbreaking” features, promising to revolutionize the field of web apps. These features are often (but not always) related to dealing with the over-engineering features already created in these frameworks previously.
Overengineering and complexity is a real concern for new developers who don’t know the difference between these frameworks and their features, or why the features exists in the first place. If you are new to this field you will feel overwhelmed, confused, and depressed by the complexity and vastness of frameworks and tools out there.
The thing to remember is this: All frameworks are built on JavaScript, and you can definitely just use pure JavaScript to create websites and apps. However, the frameworks does makes it more convenient to create apps, even though you initially have to learn the frameworks specific way of doing things.
But which to choose? Just pick one of the popular frameworks that has a big community and it will be OK. They provide more or less the same features but with different semantics, mental models, and convenience factors. They will all work fine. Just don’t pick an obscure framework where you can’t find guides or support answers on StackOverflow (even if it promises it is the best framework ever).
Here is a great annual survey on the state of JavaScript, where you can see the popularity, happiness, and usage statistics of various frameworks and tools in JavaScript: stateofjs.com
Side note: Turning Web apps into native apps
I should also mention that there are tools that makes it possible to turn your web app into Native apps on iOS and Android. I have not used these tools personally, but it is good to know that these exist, and can be an option if you want to create apps for different platforms but don’t want do deal with writing the same application in three different ways.
- Ionic: A framework for turning React, Vue, or Angular code into native apps.
- React Native: Turn React web apps into native apps.
- Vue native: Turn Vue web apps into native apps.
- Flutter: a framework by Google for building natively compiled, multi-platform applications from a single codebase.
There are more tools out there, but these are quite popular.
3. CSS, pre-processors and UI frameworks
In this chapter we take a brief look at the history and evolution of Cascading style Sheets (CSS), as well as the tools and frameworks I personally use.
What is CSS, when did it come out, and what problems did it solve?
Before CSS, every browser implemented their own way of styling web pages (how they were displayed visually). Styling was linked to browsers rather than the html documents themselves, and the styling was limited and would work differently in each browser. CSS was proposed to separate the document structure from the presentation and to standardize styling such that you could use one styling language that worked the same in all browsers. At least that was the vision, but it was not that simple.
CSS was first proposed by Håkon Wium Lie in 1994 (at the Mosaic and the Web conference), with the first official release of CSS1 coming out in 1996. Several other style sheet languages were proposed but discarded.
While browsers started implementing parts of the CSS specification (like IE 4 and Netscape 4.x), the support was incomplete and contained bugs, and CSS could therefore not be fully adopted. It was not until the end of the 1990s that some browsers (like IE 5) started achieving close to full implementation of the specification, including parts of CSS 2 which was released in 1998.
However, even if IE 5 implemented over 99% of the CSS specification, it still had bugs and some incorrect implementations. IE 5.x for windows, for example, had a flawed CSS box model compared to the specification. In addition, when bugs were later fixed, some web pages that used to work stopped working. There were also inconsistencies across browsers as some features were implemented differently in each.
These inconsistencies made it difficult for web developers to design a webpage that would look the same in all browsers. To deal with these inconsistencies, developers had to create workarounds, also known as CSS hacks and CSS filters. Individual browsers also implemented their own prefixes to some CSS parameters to avoid web pages breaking in future updates:
-moz- for Mozilla
-webkit- for Safari
-o- for Opera
-ms- for Internet Explorer and Edge
Example:
.some-class {
background: -moz-linear-gradient(..)
background: -webkit-linear-gradient(..)
background: -o-linear-gradient(..)
background: -ms-linear-gradient(..)
background: linear-gradient(..)
(The major browser vendors are moving away from vendor prefixes in favor of other methods such as @supports feature queries.)
The slow CSS adoption of some browsers also caused these browsers to get a bad reputation among developers. Yes, I am talking about Internet Explorer. Prior to IE 10, IE even supported special comment syntax such that developers could import different CSS stylesheets depending on the version of IE that was used. This way, you could provide compatibility to old browsers with old implementations.
Remember this? Happy days:
<link href="all_browsers.css" rel="stylesheet" type="text/css">
<!--[if IE]> <link href="ie_only.css" rel="stylesheet" type="text/css"> <![endif]-->
<!--[if lt IE 7]> <link href="ie_6_and_below.css" rel="stylesheet" type="text/css"> <![endif]-->
<!--[if !lt IE 7]> <![IGNORE[--><![IGNORE[]]> <link href="recent.css" rel="stylesheet" type="text/css"> <!--<![endif]-->
<!--[if !IE]--> <link href="not_ie.css" rel="stylesheet" type="text/css"> <!--<![endif]-->
Browser compatibility
In order to ensure consistency, It was important to test the website in different browsers. Tools such as BrowserStack started to appear (2011), making this easier.
And to provide compatibility for older browsers, which had old CSS engine implementations, people started creating polyfills with JavaScript. Essentially, a polyfill is a piece of code (usually JavaScript on the Web) used to provide modern functionality on older browsers that do not natively support it.
These days, the popular browsers have mostly up-to-date CSS implementations, and browser-specific CSS or polyfills are mostly not needed. To check what browsers implements a feature you want to use, you can check out caniuse.com. For example, check out the page for Flexbox compatability here.
CSS Preprocessors
Syntactically Awesome StyleSheets (Sass) appeared in 2006, created by Natalie Weizenbaum and Chris Eppstein, aimed to extend and improve CSS. Sass is a pre-processor language. For CSS this means that Sass compiles your Sass code into CSS. Sass by itself cannot be rendered in the browser.
Sass provides a bunch of useful features:
-
Variables
-
Nesting
-
Loops
-
Arguments
-
Mixins
There are other pre-processor CSS tools out there as well, but Sass is clearly the most popular.
UI Frameworks
Similar to how there are JavaScript frameworks that helps people save time writing JavaScript, there are UI frameworks that helps people saving time writing CSS. A UI framework is intended to provide pre-prepared CSS libraries (and sometimes some JS features) that can be imported and used in web development project
The most famous, and one of the oldest, is the Bootstrap UI framework, which first came out in 2011. Important features of Bootstrap include:
-
A base stylesheet with basic styling (normalization).
-
Classes for layout (grid, breakpoints, containers, etc.).
Sass for a modular and customizable experience.
-
Ready-made components (saves you lots of time).
JavaScript plugins for popups, tooltips, and more.
Icons.
Themes.
While Bootstrap is popular, there are many other UI frameworks out there. Others include Tailwind CSS and Bulma.
Modern CSS
CSS has gotten better in recent years, with built-in grids, flexbox, nesting, variables, transitions/animations, imports, and more.
It seems like the CSS specification is getting inspiration from popular CSS tools and are adding useful features every year.
Recommendations
If you want to learn more about CSS, take a look at this guide. Or browse CSS-Tricks. Or just find a guide, there are lots of great guides out there for learning CSS.
Here is my recommendation on what tools to use for UI development:
- Just use plain CSS. It is enough, especially for simple projects. Modern CSS has many of the features that you wanted from a pre-processor likeSass. And it is very convenient to not have to manage a pre-processor and compiling your Sass to CSS.
- If you feel the need for a UI framework, Bootstrap is easy to use, well maintained, and provides great looking components that you can just plug into your website or app. I have used Bootstrap in many projects, and the base stylesheet is useful even for small projects. Tailwind CSS is also a good option if you want more control of exactly how things will look. I have really enjoyed using Tailwind in some projects.
4. Bonus: Guidelines for choosing frameworks
There are many frameworks for both frontend and backend. Choosing one when you have not used them can be hard and confusing. This chapter tries to make the choosing simpler.
Components of a web app
The components of a web app are:
-
Frontend, which lives in the browser on the device that loads the website/app. The frontend creates the UI that a user interacts with. This is HTML, CSS and JavaScript.
-
Backend, which lives on a server somewhere, usually in the cloud. The backend initially sends the frontend code to the browser. The frontend then makes further requests to the backend to provide data or perform tasks. Common backend tasks are authentication and accessing data from a database.
-
Third-party services, that your app uses to add functionality or data from a third party. E.g., utilizing Google Maps in your app, or getting data from a weather service.
Frontend uses HTML, CSS and JavaScript to create a website with content and interactivity. Modern web development uses various JavaScript web frameworks and tools to make it easier to develop high quality frontends for websites and web apps.
If you need backend features, there are several options. The easiest option is to use a server-less backend service such as Firebase to run and manage a backend for you. Firebase have services for databases, authentication, notifications, and more. Another option is to rent a server and manage the backend yourself. Usually you rent a virtual machine at AWS, Azure, Google Cloud, Linode, Heroku, or similar. Then you code your backend yourself with a framework like Django (python) or Express (node.js).
In the rest of this article we will only discuss frontend web frameworks.
Frontend Web Frameworks
The list of Frontend frameworks is seemingly endless, and there seems to always be a new framework around the corner. It is a large, opinionated and complex landscape that is hard to navigate if you are new to web development.
Over the years, frontend web frameworks and the tools they depend on have become increasingly more complex, making it easier to make great apps and websites, but making it harder to understand how the web actually works.
Frontend web frameworks can be roughly categorized in the following camps:
-
JavaScript libraries and progressive frameworks that can be directly imported into your HTML file without a build step. Examples are:
jQuery
Alpine.js
Vue.js
-
Single-Page Applications (SPAs) frameworks, that require a build step (e.g., with node.js and webpack) and introduces concepts such as reactivity and components. Examples are:
Vue
React
Svelte
Angular
-
Meta/hybrid frameworks, that does the same as SPAs, but where data can be rendered on the server, aka. Server-Side Rendering (SSR), and gives even more structure of how to do things. These take on some of the backend responsibilities, but not all, and a full-scale backend framework is usually preferred for applications that are backend heavy. Examples are:
Next.js (React)
Remix (React)
Nuxt (Vue)
Angular Universal (Angular)
SvelteKit (Svelte)
Choosing a Frontend framework
First of all: There is no clear-cut answer. Even though they slightly differ in how they do things, all of these frameworks can create great apps. If you don’t know or care which framework or features you want, I recommend choosing one based on popularity/usage, community size, and enjoyment.
We will use these three metrics to find winning framework below. The data will be based on surveys, StackOverflow stats, and my own experience. The surveys in question are The State of JS 2021 Survey (2022 is not out yet) and the Stack Overflow Developer Survey (2022).
Meta/hybrid frameworks will not be considered here, as they are linked to the frontend framework of choice (Next.js or Remix for React, Nuxt for Vue, Angular Universal for Angular, SvelteKit for Svelte).
Usage numbers
According to both The State of JS 2021 and the Stack Overflow Developer Survey (2022), React is currently the most used frontend web framework, and has enjoyed that spot for many years. React came out in 2013, the first of these modern web frameworks.
In The State of JS survey, we have Angular, Vue.js, and Svelte in descending order after React. In this survey they did not consider jQuery as a framework, so it is not listed.
In the Stack Overflow Developer Survey, we can see that jQuery is number two behind React, and then Angular, Vue and Svelte. Even if jQuery is by many considered an old library, is still widely used in the web today, but this is mostly for websites with simple UIs (for which it is a good choice, IMO). Note that this comparison includes backend frameworks and runtimes as well.
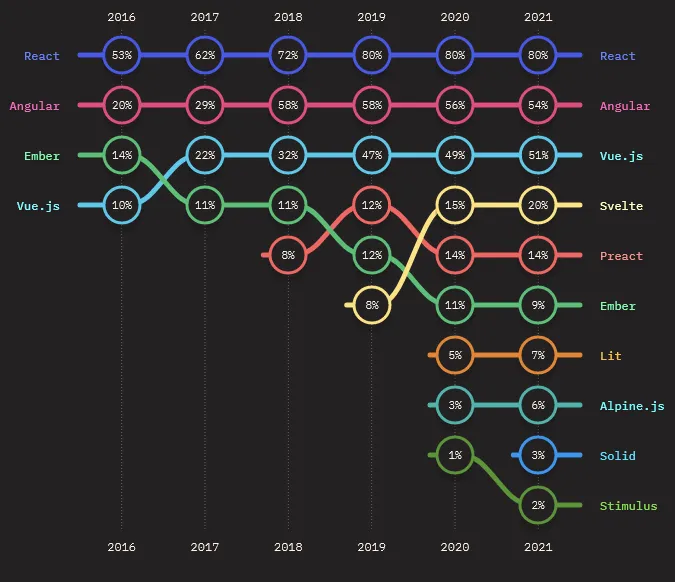
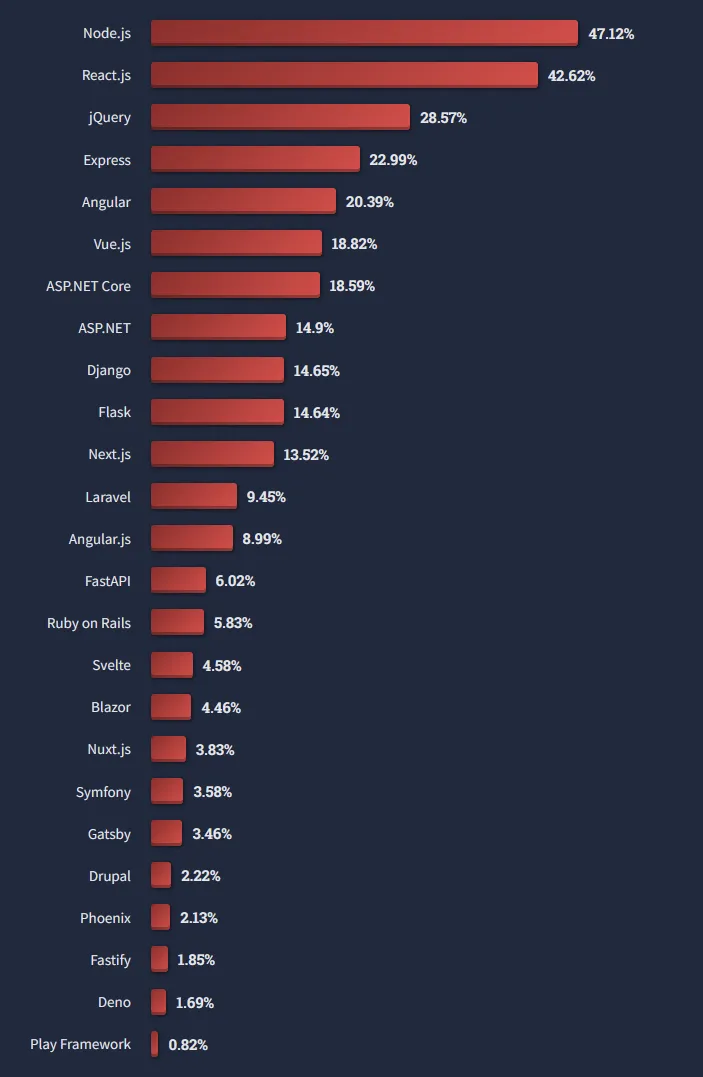
Community numbers
Here we will use numbers from Github and Stack Overflow (If you have a suggestion for a better way to gauge this, let me know in the comments).
The amount of questions on Stack Overflow will give an indication of how easy it is to find answers when you run into problems.
React:
Released 2013
Maintained by Meta
200k stars on Github
-
431,729 questions tagged with react on Stack Overflow
Vue:
Released 2014
-
Maintained by Evan You and the rest of the Vue team.
200k stars on Github
-
100,095 questions tagged with Vue on Stack Overflow
Angular:
Released 2016
Maintained by Google
85k stars on Github
-
288,323 questions tagged with Angular on Stack Overflow
Svelte:
Released 2016
-
Maintained by Rich Harris, Simon Holthausen, and others at Vercel.
63k stars on Github
-
4,027 questions tagged with Svelte on Stack Overflow
jQuery
Released 2006
-
Maintained by The jQuery team
57k stars on Github
-
1,031,599 questions tagged with jQuery on Stack Overflow
The amount of jQuery questions on Stack Overflow speaks of its high usage.
Enjoyment
Lets first see what the survey says. The State of JS Survey used a Positive/negative split format, and the Stack Overflow Developer Survey used a Loved vs. Dreaded format:
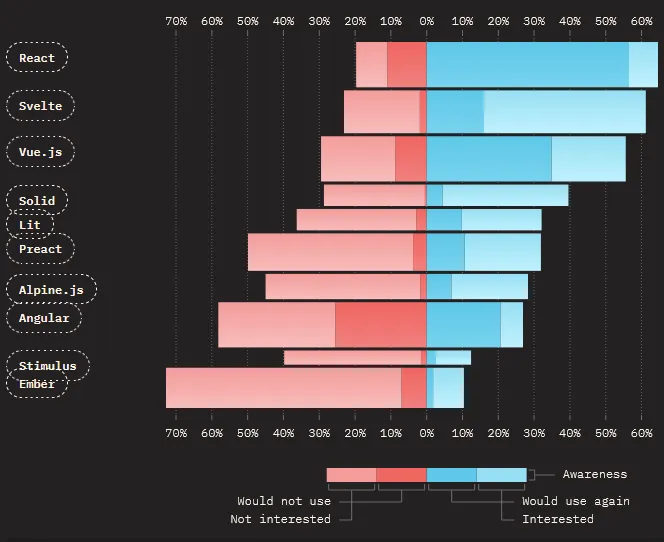
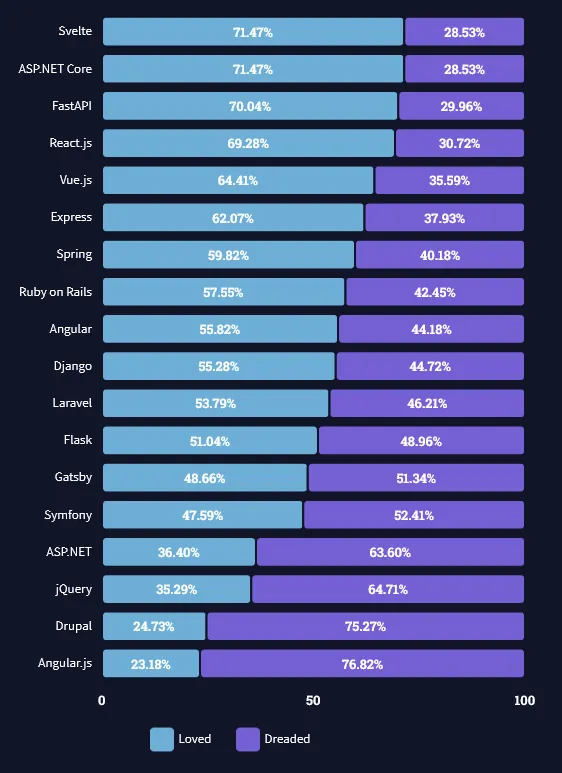
In both of these, React and Svelte comes out at the top, with Vue slightly behind.
In my experience, enjoyment of a web framework comes from simplicity, ease learning and using, and being able to quickly code up a project without jumping through too many hoops or having to google too many things.
I recently learned Svelte and have used it in one project. I liked both how easy it was to understand its way of doing things, and its simple build process and configuration.
I have also used React, and I liked how many resources there was on the web for learning it. For every question I had there was an article, video, or an answer ready on Stack Overflow.
I have also used Vue extensively and enjoy its declarative syntax and that it is a progressive framework. There are also many resources on the web for learning Vue.
I am very surprised that jQuery is so dreaded. I find that it is a great library to use when I just want to code something simple that doesn’t need all the firepower that the other modern frameworks has (like reactive states, data store, frontend routing, etc.). Just pop the CDN link for jQuery in the header tag and I’m ready to go!
To sum up. I think all of the ones I mentioned above is great for making UIs. If I was a new web developer with no experience, I would probably go with React due to its big community and vast learning resources available online. Svelte would be my second choice because its simplicity is so nice. And for simple websites I would definitely just use jQuery.
I haven’t tried Angular, so I can’t give any advice on that, but from people I have talked to it seems like individual developers thinks it has too strict rules, while large enterprises likes it because it enforces a certain way of doing things. For enterprises, this makes it easier to maintain large code-bases.
Choosing Frontend stack based on website requirements
In this section we will try to think about how different Frontend web stacks fits different kinds of apps and websites. Starting with a simple static website and building up to a complex modern web app.
We haven’t discussed CSS frameworks. So I am just going to add suggestions for ones I like: TailwindCSS and Bootstrap. I recommend TailwindCSS for most projects. It is a simplistic CSS framework that provides convenience and enjoyment, while not standardizing how the way websites/apps look and feel. Bootstrap is something I use for simple projects where I just want to import a CSS toolkit with a header link and get going.
Basic static site with no or limited interactivity
This is like your local hairdresser type website. These kind of websites are mostly static, with rare updates, and limited amount of UI interactivity.
While something like React would definitely work for this website, it is overkill and would mostly just complicate things. You should only use an advanced framework for this if you already have a good workflow with it.
Suggested stack:
jQuery/Alpine.js + Bootstrap
Just create an HTML5 file, import the jQuery and Bootstrap CDN links, and you are ready to go!
A website with an app-like UI, and no need for SEO
Any website where you expect the user to interact with the site.
Suggested stack:
React + TailwindCSS
Requires a bit of setup, but it is a powerful combination once you get going. Many large and popular websites and apps use React.
Alternatively use Vue, Svelte, or Angular instead of React.
An app-like website, but you have content that needs SEO
This may be any website that uses a frontend web framework like React, but which has content that it wants a search engine crawlers to find. React, Angular, Vue and Svelte are all Single-Page Applications (SPAs), which is bad for SEO, so for this situation we need a Meta/Hybrid framework where Server-Side Rendering can be used.
Suggested stack:
Next.js (React) + TailwindCSS
Or alternatively use Nuxt (for Vue), SvelteKit (for Svelte), or Angular Universal (for Angular).
Conclusion
This post has evaluated frontend frameworks and here is my recommendation:
-
Overall winner: React and Next.js + TailwindCSS
-
React is a JavaScript frontend framework for making highly interactive UIs.
-
TailwindCSS is a utility-first CSS framework for rapid UI development.
-
Use Next.js (a React meta-framework) if you need server-side rendering (SSR), e.g., for Search-Engine Optimization (SEO).
-
For websites that needs any sort of interactive UI (which includes most modern websites and apps), React is a good choice. It has been the most used javascript frontend web framework for at least five years and the community is huge.
-
-
Alternative for very simple websites: jQuery / Alpine.js + Bootstrap
-
jQuery is a JavaScript library designed to simplify HTML DOM tree traversal and manipulation, as well as event handling, CSS animation, and Ajax. It has an easy-to-use API that works across a multitude of browsers. Installing jQuery is easy, just add a script tag with a CDN link in your HTML document.
-
Alpine.js is a minimal tool for composing behavior and interactive UIs directly in your markup. Think of it like jQuery for the modern web. Installing it is as easy as jQuery, just pop in a script tag and get going.
-
Bootstrap is a CSS toolkit with grids, ready-made components, icons, and more. They have a CDN version that can be included directly into the HTML document.
-
For very simple websites/apps, React can be a little bit cumbersome. jQuery and alpine.js can be directly imported into the HTML document and you can get going with a very simple setup.
jQuery is still used on the majority of all websites, and used by 29.21% of professional developers (placed after react at 44.31%).